Python is popular programming language that has lot of libraries available to perform various tasks. One such task is downloading videos from YouTube. In this article we will discuss how to write a Python program to download YouTube videos using the pytube library.
Create Music Player in Python with Output
Any Language Translator in Python
Step by step Video Python Program to Download Youtube Videos
Installing the Required Libraries
Before we start we need to install the required libraries. We will be using following libraries:
!pip install Progressbar
from tkinter import *
from tkinter import messagebox
from pytube import YouTube
from tqdm import tqdm
tkinter
is Python GUI library.messagebox
is module intkinter
that provides a set of standard dialog boxes.pytube
is Python library for downloading YouTube videos.tqdm
is library for displaying progress bars in the command line
Complete Code:
from tkinter import *
from tkinter import messagebox
from pytube import YouTube
from tqdm import tqdm
def get_video():
global streams
# get the video link from the entry widget
video_link = entry.get()
try:
# create a YouTube object
yt = YouTube(video_link)
# get the available video streams
streams = yt.streams.filter(file_extension="mp4").order_by('resolution').desc()
# populate the listbox with the available resolutions
listbox.delete(0, END)
for i, stream in enumerate(streams):
listbox.insert(END, "{}. {}p".format(i+1, stream.resolution))
except:
messagebox.showerror("Error", "Invalid video link.")
def download_video():
# get the selected video stream from the listbox
resolution_choice = listbox.curselection()[0]
global video
video = streams[resolution_choice]
# create a progress bar
global progress
progress = Progressbar(window, orient=HORIZONTAL, length=300, mode='determinate')
progress.pack()
# create a label to display the time remaining
global time_label
time_label = Label(window, text="")
time_label.pack()
# download the video and update the progress bar
video_path = video.download(filename=video.title, output_path=".")
progress['value'] = 100
time_label.config(text="Download complete.")
messagebox.showinfo("Download Complete", "The video has been downloaded successfully.")
def update_progress(stream, chunk, bytes_remaining):
size = stream.filesize
downloaded = size - bytes_remaining
progress_value = int(downloaded / size * 100)
progress['value'] = progress_value
time_remaining = int(bytes_remaining / stream.file.speed)
time_label.config(text="Time remaining: {} seconds".format(time_remaining))
# create the main window
window = Tk()
window.title("YouTube Downloader")
# create an entry widget for the video link
link_label = Label(window, text="Video Link:")
link_label.pack()
entry = Entry(window, width=50)
entry.pack()
# create a button to get the available video streams
button = Button(window, text="Get Video", command=get_video)
button.pack()
# create a listbox to display the available video streams
listbox_label = Label(window, text="Available Resolutions:")
listbox_label.pack()
listbox = Listbox(window)
listbox.pack()
# create a button to download the selected video stream
download_button = Button(window, text="Download", command=download_video)
download_button.pack()
# start the main loop
window.mainloop()
- The function
get_video
is called when tuser clicks the “Get Video” button. global streams
is used to makestreams
variable available to other functions.- The function gets YouTube video link entered by the user in the entry widget.
- A
YouTube
object is created with video link. - The
filter()
method is used to get available video streams with the file extension of “mp4”. Theorder_by()
method is used to sort the streams by resolution in descending order. - The function populates listbox with available resolutions by deleting the previous items in the listbox and inserting new items with the
insert()
method. Theenumerate()
function is used to add a numbering system to the resolutions.
- The function
download_video
is called when user clicks the “Download” button. - The
curselection()
method is used to get index of the selected resolution in the listbox. global video
is used to makevideo
variable available to other functions.- The function creates a progress bar and a label to display the time remaining.
- The
download()
method is used to download the video and progress bar is updated as the video is downloading. - Once the download is complete progress bar is set to 100 and the time label is updated to show that the download is complete. Finally, a message box is displayed to inform the user that the download is complete.
- Inside the
get_video()
function atry-except
block is used to catch any exceptions that may occur while creating aYouTube
object from the video link entered by the user. If the video link is invalid, an error message is displayed using themessagebox.showerror()
method. - If the video link is valid then available video streams are obtained by filtering the streams based on their file extension and ordering them based on their resolution. The obtained streams are stored in the
streams
global variable. - The
listbox
widget is then cleared using thelistbox.delete()
method and the available resolutions are displayed in thelistbox
widget usinglistbox.insert()
method. The format of the resolution displayed is “{stream_number}. {resolution}p”, where thestream_number
is the index of the stream in thestreams
list plus one, and theresolution
is the resolution of the stream. - The
download_video()
function is called when user clicks the “Download” button. The function first gets the index of the selected stream from thelistbox
widget using thelistbox.curselection()
method. The selected stream is then stored in thevideo
global variable. - A
Progressbar
widget is created to display progress of the video download. TheProgressbar
widget is oriented horizontally and has a length of 300 pixels. The progress bar is then packed into the main window using thepack()
method. - A
Label
widget is created to display time remaining for the download to complete. TheLabel
widget is then packed into the main window using thepack()
method. - The video download is initiated by calling the
download()
method of selected stream object. The video is saved with a filename equal to the title of the video and saved in the current directory. As the video download progresses, theupdate_progress()
function is called to update the progress bar and the time remaining label. When the download is complete, a message is displayed using themessagebox.showinfo()
method. - The
update_progress()
function is defined to update progress bar and time remaining label during the video download. The function takes in thestream
,chunk
, andbytes_remaining
parameters. Thesize
of the file being downloaded is obtained from thestream.filesize
attribute. The amount of data downloaded so far is calculated by subtracting thebytes_remaining
from thesize
. The progress bar value is calculated as a percentage of the downloaded data over the total size of the file. The time remaining is calculated by dividing thebytes_remaining
by the speed of the download. - Finally main window is created using Tk() constructor and various widgets such as labels entry, listbox, buttons progress bar, and label are added to it using the Label(), Entry(), Listbox(), Button(), Progressbar(), and Label() constructors, respectively. The pack() method is used to add the widgets to the window. mainloop() method is called to run the main event loop of the window.
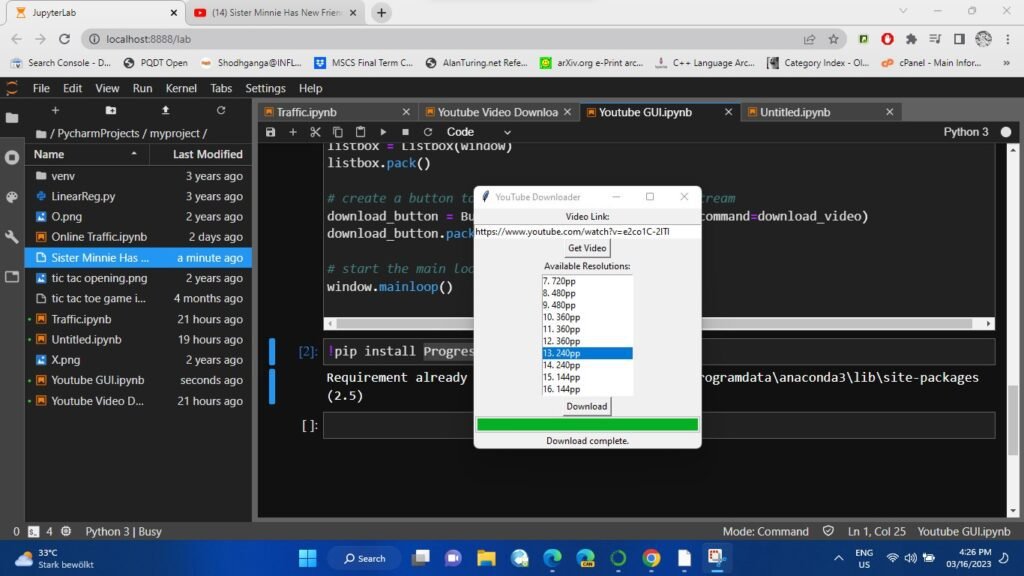
Here are some useful links related to downloading YouTube videos with Python:
- The official documentation of the pytube library: https://python-pytube.readthedocs.io/en/latest/
- The GitHub repository for pytube, where you can find source code and examples: https://github.com/pytube/pytube
- The documentation for the Python Standard Library’s
tkinter
module, which is used in the example code: https://docs.python.org/3/library/tkinter.html - The documentation for the
tqdm
library, which provides a progress bar for downloads: https://tqdm.github.io/ - A tutorial on using pytube to download YouTube videos: https://www.geeksforgeeks.org/python-program-to-download-complete-youtube-playlist-without-using-any-third-party-library/