Python Program to Download YouTube Videos
Here’s a step-by-step Python Program to Download YouTube videos using Python and Google Colab:
- First you will need to sign in to Google Colab with your Google account. Google Colab is free online environment for running Python code and comes with pre-installed libraries like PyTube that we’ll use for downloading YouTube videos.
- Open a new Python 3 notebook in Google Colab by going to “File” -> “New Notebook” -> “Python 3”.
- Import necessary libraries for our YouTube video download manager: PyTube, regex, and IPython.display. You can import these libraries by adding the following code to a code cell in your notebook
import re
import ipywidgets as widgets
from IPython.display import display
from pytube import YouTube
Next we’ll define a function for downloading YouTube video. This function will take in YouTube video link and return the filename of the downloaded video. We’ll use regular expressions to extract the video ID from the YouTube video link, and then download the video in the highest possible resolution using PyTube. You can define this function by adding following code to a code cell in your notebook:
def download_video(video_link):
try:
# Extract the video ID from the video link
regex = r"(?:\/|%3D|v=|vi=)([0-9A-Za-z_-]{11})(?:[%#?&]|$)"
match = re.search(regex, video_link)
if match:
video_id = match.group(1)
else:
raise ValueError("Invalid YouTube video link")
# Download the YouTube video in the highest possible resolution
yt = YouTube(f"https://www.youtube.com/watch?v={video_id}")
stream = yt.streams.get_highest_resolution()
filename = stream.default_filename
stream.download()
return filename
except (ValueError, RegexMatchError, Exception):
return False
Now we will define function to handle the download button click event. This function will get YouTube video link from the text input field, download the video using the download_video
function, and then show a success or error message to the user. You can define this function by adding the following code to a code cell in your notebook:
def on_download_button_click(b):
# Get the YouTube video link from the text input field
video_link = text_input.value
# Download the YouTube video and show a success or error message to the user
filename = download_video(video_link)
if filename:
success_label.value = f"Download successful! Video saved as {filename}."
else:
success_label.value = "Invalid or unsupported YouTube video link."
Here is a Video python program to download youtube videos
Complete Python Program to Download YouTube Videos
import re
import ipywidgets as widgets
from IPython.display import display
from pytube import YouTube
# Define a function to download a YouTube video
def download_video(video_link):
try:
# Extract the video ID from the video link
regex = r"(?:\/|%3D|v=|vi=)([0-9A-Za-z_-]{11})(?:[%#?&]|$)"
match = re.search(regex, video_link)
if match:
video_id = match.group(1)
else:
raise ValueError("Invalid YouTube video link")
# Download the YouTube video in the highest possible resolution
yt = YouTube(f"https://www.youtube.com/watch?v={video_id}")
stream = yt.streams.get_highest_resolution()
stream.download()
return True
except (ValueError, RegexMatchError, Exception):
return False
# Define a function to handle the download button click event
def on_download_button_click(b):
# Get the YouTube video link from the text input field
video_link = text_input.value
# Download the YouTube video and show a success or error message to the user
success = download_video(video_link)
if success:
success_label.value = "Download successful!"
else:
success_label.value = "Invalid or unsupported YouTube video link."
# Create a text input field for the user to input the YouTube video link
text_input = widgets.Text(
placeholder='https://www.youtube.com/watch?v=Qu1fBeX9vE4&t=50s&ab_channel=AadhiBaat',
description='Link:',
layout=widgets.Layout(width='50%')
)
# Create a download button
download_button = widgets.Button(
description='Download',
layout=widgets.Layout(width='auto', height='auto')
)
# Attach the download button click event handler
download_button.on_click(on_download_button_click)
# Create a label to show a success or error message to the user
success_label = widgets.Label(
value='',
layout=widgets.Layout(margin='10px 0')
)
# Display the GUI components
display(text_input)
display(download_button)
display(success_label)
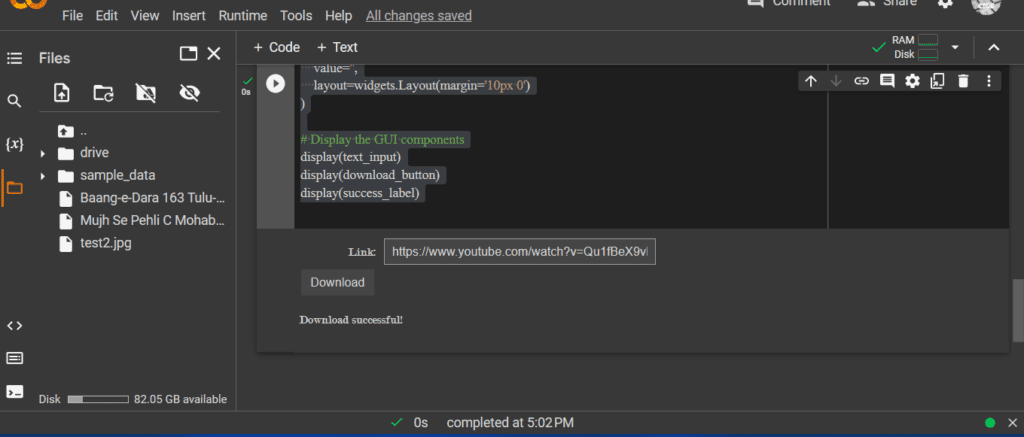
Colab Link to Download Code:
https://colab.research.google.com/drive/1xn5Zfblbzlx7keHOqt5xLZb7R-6vZgoA?usp=sharing
Useful Links
here are some useful links related to creating a YouTube video download manager using Python and Google Colab:
- Google Colab: https://colab.research.google.com/notebooks/intro.ipynb
- Pytube documentation: https://python-pytube.readthedocs.io/en/latest/
- Regular expressions in Python: https://docs.python.org/3/library/re.html
- YouTube video download API: https://developers.google.com/youtube/v3/docs/videos/download
- Video formats and quality options supported by Pytube: https://python-pytube.readthedocs.io/en/latest/user/streams.html
I hope these links are helpful in your learning journey!