here’s a step-by-step article on how to create a Python program to compress an image using tkinter and PIL.
Python program to Compress image
Images are an integral part of modern computing, but they can often be quite large in size, making them difficult to work with. Fortunately, there are many tools available that can help you reduce the size of your images, making them easier to work with and faster to load. In this tutorial, we’ll show you how to create a Python program to compress an image using the tkinter and PIL libraries.
Video For Python Program to Compress an Image
Also Learn:
Python Program to Convert any Video into MP3 – MP4 to MP3 in Python
Create a News Feed Application in Python | Python Project
Python Program to Handle Missing Values in Data
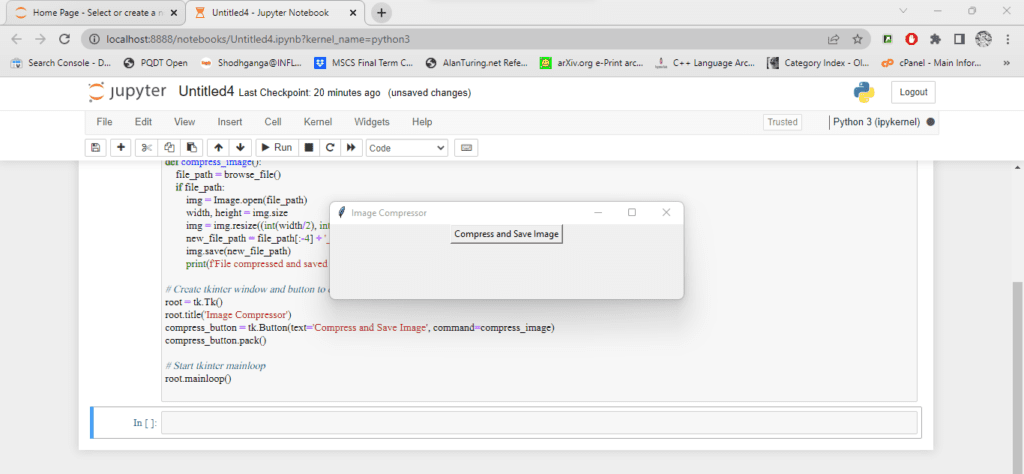
Step 1: Install tkinter and PIL
The first step is to install the required libraries. tkinter is a GUI toolkit for Python, and PIL (Python Imaging Library) is a library that adds support for opening, manipulating, and saving many different image file formats.
You can install these libraries using pip, the package manager for Python:
pip install tkinter
pip install pillow
Step 2: Import the required libraries
Once you have installed tkinter and PIL, you need to import them into your Python program:
import tkinter as tk
from tkinter import filedialog
from PIL import Image
Step 3: Create a function to browse for the image file
Next, you need to create a function that allows the user to browse for the image file they want to compress. This function will use the filedialog
module from tkinter to display a file dialog window, where the user can select the image file they want to compress.
def browse_file():
file_path = filedialog.askopenfilename()
return file_path
The askopenfilename()
method displays a file dialog window that allows the user to select a file. It returns the file path of the selected file.
Step 4: Create a function to compress and save the image
Now that you have a function to browse for the image file, you need to create a function that compresses and saves the image. This function will use the Image
module from PIL to open the image file, resize it to half its original size, and then save the compressed image to a new file.
def compress_image():
file_path = browse_file()
if file_path:
img = Image.open(file_path)
width, height = img.size
img = img.resize((int(width/2), int(height/2)))
new_file_path = file_path[:-4] + '_compressed.jpg'
img.save(new_file_path)
print(f'File compressed and saved as {new_file_path}')
The compress_image()
function first calls the browse_file()
function to get the file path of the image to compress. It then uses the Image.open()
method to open the image file, and the resize()
method to resize the image to half its original size. Finally, it saves the compressed image to a new file using the save()
method, and prints a message to the console indicating the location of the compressed image file.
Step 5: Create the GUI
Now that you have the functions to browse for the image file and compress and save the image, you need to create the GUI that allows the user to interact with the program.
root = tk.Tk()
root.title('Image Compressor')
compress_button = tk.Button(text='Compress and Save Image', command=compress_image)
compress_button.pack()
root.mainloop()
Sure, here’s a step-by-step article on how to create a Python program to compress an image using tkinter and PIL.
Python program to Compress image
Images are an integral part of modern computing, but they can often be quite large in size, making them difficult to work with. Fortunately, there are many tools available that can help you reduce the size of your images, making them easier to work with and faster to load. In this tutorial, we’ll show you how to create a Python program to compress an image using the tkinter and PIL libraries.
Step 1: Install tkinter and PIL
The first step is to install the required libraries. tkinter is a GUI toolkit for Python, and PIL (Python Imaging Library) is a library that adds support for opening, manipulating, and saving many different image file formats.
You can install these libraries using pip, the package manager for Python:
Copy codepip install tkinter
pip install pillow
Step 2: Import the required libraries
Once you have installed tkinter and PIL, you need to import them into your Python program:
pythonCopy codeimport tkinter as tk
from tkinter import filedialog
from PIL import Image
Step 3: Create a function to browse for the image file
Next, you need to create a function that allows the user to browse for the image file they want to compress. This function will use the filedialog
module from tkinter to display a file dialog window, where the user can select the image file they want to compress.
pythonCopy codedef browse_file():
file_path = filedialog.askopenfilename()
return file_path
The askopenfilename()
method displays a file dialog window that allows the user to select a file. It returns the file path of the selected file.
Step 4: Create a function to compress and save the image
Now that you have a function to browse for the image file, you need to create a function that compresses and saves the image. This function will use the Image
module from PIL to open the image file, resize it to half its original size, and then save the compressed image to a new file.
pythonCopy codedef compress_image():
file_path = browse_file()
if file_path:
img = Image.open(file_path)
width, height = img.size
img = img.resize((int(width/2), int(height/2)))
new_file_path = file_path[:-4] + '_compressed.jpg'
img.save(new_file_path)
print(f'File compressed and saved as {new_file_path}')
The compress_image()
function first calls the browse_file()
function to get the file path of the image to compress. It then uses the Image.open()
method to open the image file, and the resize()
method to resize the image to half its original size. Finally, it saves the compressed image to a new file using the save()
method, and prints a message to the console indicating the location of the compressed image file.
Step 5: Create the GUI
Now that you have the functions to browse for the image file and compress and save the image, you need to create the GUI that allows the user to interact with the program.
pythonCopy coderoot = tk.Tk()
root.title('Image Compressor')
compress_button = tk.Button(text='Compress and Save Image', command=compress_image)
compress_button.pack()
root.mainloop()
The tk.Tk()
method creates a new tkinter window, and the title()
method sets the title of the window. The tk.Button()
method creates a new button with the label “Compress and Save Image”, and the command=
parameter specifies that
when the user clicks the button, the compress_image()
function will be executed.
The compress_button.pack()
method adds the button to the tkinter window, and the root.mainloop()
method starts the tkinter event loop, which waits for the user to interact with the GUI.
Final Code
Here is the complete code:
import tkinter as tk
from tkinter import filedialog
from PIL import Image
# Define function to browse for image file
def browse_file():
file_path = filedialog.askopenfilename()
return file_path
# Define function to compress image and save to new file
def compress_image():
file_path = browse_file()
if file_path:
img = Image.open(file_path)
width, height = img.size
img = img.resize((int(width/2), int(height/2)))
new_file_path = file_path[:-4] + '_compressed.jpg'
img.save(new_file_path)
print(f'File compressed and saved as {new_file_path}')
# Create tkinter window and button to compress and save image
root = tk.Tk()
root.title('Image Compressor')
compress_button = tk.Button(text='Compress and Save Image', command=compress_image)
compress_button.pack()
# Start tkinter mainloop
root.mainloop()
Here are some useful links related to this topic: