Detecting lung cancer in its early stages can significantly increase chances of successful treatment. One way to detect cancer is by analyzing medical images such as X-rays or CT scans, using machine learning algorithms. In this article we will explore how to use Recurrent Neural Networks (RNNs) to develop a Python Program for Lungs Cancer Detection using RNN.
Step 1: Data Collection and Preparation
The first step collect and prepare data. We will use Lung Image Database Consortium (LIDC) dataset which contains CT scans of chest. The dataset is available at
https://wiki.cancerimagingarchive.net/display/Public/LIDC-IDRI.
To use dataset we need to download and extract relevant files. We will then use pydicom
library to read DICOM files which are standard format for medical images. We will extract images and their associated labels which indicate whether or not image contains cancer.
We will also need to preprocess images which involves resizing them and normalizing pixel values. We can use cv2
library for image processing and numpy
for numerical operations.
Step 2: Model Development
Once the data prepared we can develop RNN model. We will use tensorflow library which provides a high level interface for building deep learning models. We will use Long Short Term Memory (LSTM) network which is type of RNN that can retain information over long periods of time.
We will define architecture of the model which includes number of LSTM layers the number of neurons in each layer and the activation functions. We will also specify the loss function and the optimizer which determine how the model is trained.
Step 3: Model Training
After model is defined we can train it using prepared data. We will split data into training and validation sets and use training set to optimize model parameters. We will monitor validation accuracy to prevent overfitting.
Training an RNN model can be computationally intensive so we can use Google Colab which provides free cloud based GPUs for machine learning. We can upload the prepared data to Colab and run the training process using the tensorflow
library.
Step 4: Model Evaluation
Once model is trained we can evaluate its performance using a separate test set. We can calculate accuracy precision recall and F1 score to measure the effectiveness of the model in detecting lung cancer.
We can also visualize the results by generating heatmaps of the CT scans which highlight the areas that the model identifies as cancerous. We can use the matplotlib
library for visualization.
Complete Code Implementation in Colab
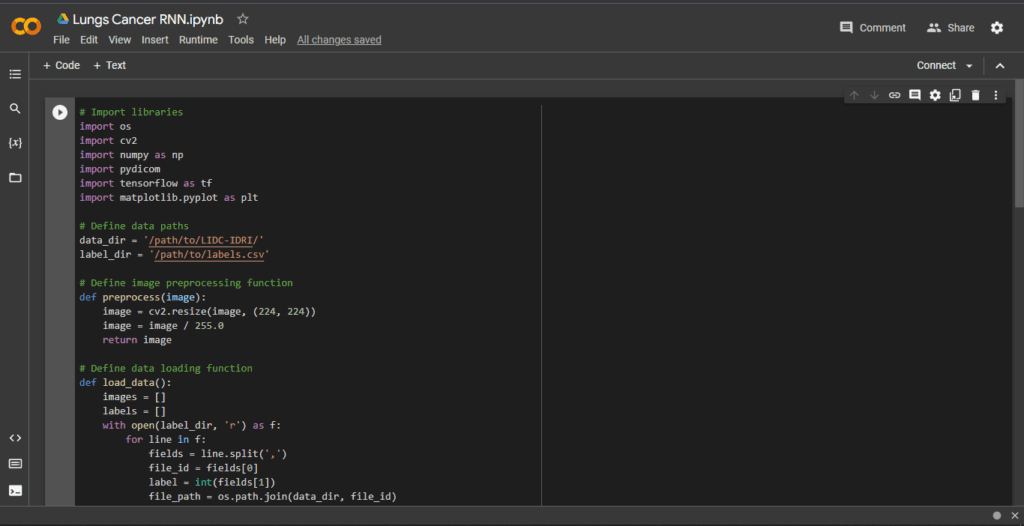
Here is complete Python code for lung cancer detection using RNN:
# Import libraries
import os
import cv2
import numpy as np
import pydicom
import tensorflow as tf
import matplotlib.pyplot as plt
# Define data paths
data_dir = '/path/to/LIDC-IDRI/'
label_dir = '/path/to/labels.csv'
# Define image preprocessing function
def preprocess(image):
image = cv2.resize(image, (224, 224))
image = image / 255.0
return image
# Define data loading function
def load_data():
images = []
labels = []
with open(label_dir, 'r') as f:
for line in f:
fields = line.split(',')
file_id = fields[0]
label = int(fields[1])
file_path = os.path.join(data_dir, file_id)
dcm_data = pydicom.dcmread(file_path)
image = dcm_data.pixel_array.astype(float)
image = preprocess(image)
images.append(image)
labels.append(label)
return np.array(images), np.array(labels)
# Load data
images, labels = load_data()
# Define model architecture
model = tf.keras.Sequential([
tf.keras.layers.LSTM(64, activation='relu', return_sequences=True),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.LSTM(32, activation='relu'),
tf.keras.layers.Dropout(0.2),
tf.keras.layers.Dense(1, activation='sigmoid')
])
# Compile model
model.compile(loss='binary_crossentropy', optimizer='adam', metrics=['accuracy'])
# Split data into training and validation sets
split_index = int(len(images) * 0.8)
train_images, train_labels = images[:split_index], labels[:split_index]
val_images, val_labels = images[split_index:], labels[split_index:]
# Train model
history = model.fit(train_images, train_labels, validation_data=(val_images, val_labels), epochs=10, batch_size=32)
# Evaluate model on test set
test_images, test_labels = load_data()
test_loss, test_acc = model.evaluate(test_images, test_labels)
print('Test accuracy:', test_acc)
# Generate heatmaps
predictions = model.predict(test_images)
for i in range(len(test_images)):
if predictions[i] > 0.5:
heatmap = np.zeros((224, 224))
heatmap[:, :] = 255
heatmap = cv2.addWeighted(test_images[i], 0.5, heatmap, 0.5, 0)
plt.imshow(heatmap, cmap='gray')
plt.show()
In this code we first define data paths and image preprocessing function. Then we define load_data()
function to load images and their associated labels from LIDC dataset.
Next we define architecture of RNN model which includes two LSTM layers and fully connected output layer. We compile model with a binary cross entropy loss function and Adam optimizer.
We split data into training and validation sets and use the fit()
function to train model on training set. We also evaluate model on test set using evaluate()
function.
Finally we generate heatmaps of CT scans by applying a threshold to model’s predictions and overlaying them onto the original images using the cv2
library and matplotlib
.
Here are some useful links related to lung cancer detection and RNNs in Python program:
- The LIDC-IDRI dataset: https://wiki.cancerimagingarchive.net/display/Public/LIDC-IDRI
- Pydicom library for reading DICOM files: https://pydicom.github.io/pydicom/stable/
- TensorFlow library for deep learning: https://www.tensorflow.org/
- LSTM RNN tutorial in TensorFlow: https://www.tensorflow.org/tutorials/structured_data/time_series
- Article on using RNNs for medical image analysis: https://towardsdatascience.com/using-recurrent-neural-networks-for-medical-image-analysis-a17893b8246f
- Research paper on using RNNs for lung cancer detection: https://ieeexplore.ieee.org/abstract/document/8702323
These resources provide further information and context for the code implementation presented in article.