If you are website owner or digital marketer you know the importance of driving organic traffic to your website. Organic traffic is traffic that comes to your website from search engines, social media, and other sources without any paid promotion. In this tutorial we will show you how to create Website Traffic Bot in Python to generate traffic for website by sending requests and impressions to the website using Selenium and Requests libraries.
Video For Website Traffic Bot in Python
Import the Required Libraries
The first step is to import required libraries:
requests
random time
selenium.webdriver selenium.webdriver.chrome.service
selenium.webdriver.chrome.options.
The requests library is used to send HTTP requests to website. The random library is used to generate random user agents and referrers. The time library is used to add a delay between requests. The selenium.webdriver library is used to automate the browser actions, while selenium.webdriver.chrome.service and selenium.webdriver.chrome.options are used to configure the Chrome browser.
import requests
import random
import time
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
Define Website URL and Impressions
In this step we define the website URL that we want to send traffic to and the number of visits and impressions per visit. We ask user to input the number of visits and impressions per visit. We will use these variables later in loop to simulate traffic to the website.
website_url = "https://programmerschallenge.tech"
num_visits = int(input("Enter the number of visits: "))
num_impressions = int(input("Enter the number of impressions per visit: "))
Define User Agents and Referrers
In this step we define the user agents and referrers that we will use to simulate organic traffic. User agents are used to identify browser and operating system of user. Referrers are used to identify the source of traffic. We define a list of user agents and referrers and use random.choice() function to select a random user agent and referrer for each visit.
user_agents = ["Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/94.0.4606.81 Safari/537.36", "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/15.0 Safari/605.1.15", "Mozilla/5.0 (iPhone; CPU iPhone OS 15_0 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/15 Mobile/15E148 Safari/604.1"]
referrers = ["https://www.google.com/search?q=facebook", "https://www.bing.com/search?q=website+traffic+bot", "https://www.facebook.com/", "https://www.twitter.com/", "https://www.reddit.com/r/webdev"]
Configure the Chrome Browser
In this step we configure the Chrome browser using selenium.webdriver.chrome.options. We add two options to ChromeOptions object: “–no-sandbox” and “–disable-dev-shm-usage”. These options prevent Chrome browser from using the sandbox and shared memory which can cause issues when running the browser in a container. We also create a webdriver object using chromedriver executable and the ChromeOptions object.
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument('--no-sandbox')
chrome_options.add_argument('--disable-dev-shm-usage')
driver = webdriver.Chrome(executable_path='C:\chromedriver_win32\chromedriver.exe', chrome_options=chrome_options)
Loop through the number of visits specified by the user. For each visit choose a random user agent and referrer and create session with user agent and referrer headers.
for i in range(num_visits):
user_agent = random.choice(user_agents)
referrer = random.choice(referrers)
session = requests.Session()
session.headers.update({"User-Agent": user_agent, "Referer": referrer})
Inside the loop for impression script will first generate random scroll position on page using JavaScript. It does this by calling the execute_script
method on the driver object, passing in a JavaScript command as a string. The JavaScript command generates a random number between 0 and the height of the web page (i.e., the scrollable height) using document.body.scrollHeight
and assigns it to scroll_position
variable.
The script then scrolls page to generated position using same execute_script
method with a different JavaScript command. The command uses scrollTo
method to scroll page horizontally and vertically to the given coordinates. In this case it scrolls vertically to the randomly generated scroll_position
.
Finally script waits for a random amount of time between 2 and 10 seconds using time.sleep
method before printing the current impression number and scroll position.
# Loop through the number of impressions per visit
for j in range(num_impressions):
# Scroll to a random position on the page using JavaScript
scroll_position = random.randint(0, driver.execute_script("return document.body.scrollHeight"))
driver.execute_script(f"window.scrollTo(0, {scroll_position})")
# Wait for a random time between 2 and 10 seconds before scrolling again
time.sleep(random.randint(2, 10))
# Print the current impression number and scroll position
print(f"Impression {j+1}: Scroll position {scroll_position}")
Complete Code
# Install required packages
!pip install selenium
!apt-get update
!apt install chromium-chromedriver
# Import libraries
import requests
import random
import time
from selenium import webdriver
from selenium.webdriver.chrome.service import Service
from selenium.webdriver.chrome.options import Options
# Define website url as input
website_url = "https://haidermobiles.com/vivo-y20-price-in-pakistan"
# Define number of visits and impressions per visit
num_visits = int(input("Enter the number of visits: "))
num_impressions = int(input("Enter the number of impressions per visit: "))
# Define user agents and referrers to simulate organic traffic
user_agents = ["Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/94.0.4606.81 Safari/537.36", "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/15.0 Safari/605.1.15", "Mozilla/5.0 (iPhone; CPU iPhone OS 15_0 like Mac OS X) AppleWebKit/605.1.15 (KHTML, like Gecko) Version/15 Mobile/15E148 Safari/604.1"] # You can add more user agents here
referrers = ["https://www.google.com/search?q=facebook", "https://www.bing.com/search?q=website+traffic+bot", "https://www.facebook.com/", "https://www.twitter.com/", "https://www.reddit.com/r/webdev"] # You can add more referrers here
chrome_options = webdriver.ChromeOptions()
chrome_options.add_argument('--no-sandbox')
chrome_options.add_argument('--disable-dev-shm-usage')
driver = webdriver.Chrome(executable_path='C:\chromedriver_win32\chromedriver.exe', chrome_options=chrome_options)
# Loop through the number of visits
for i in range(num_visits):
# Choose a random user agent and referrer
user_agent = random.choice(user_agents)
referrer = random.choice(referrers)
# Create a session with the user agent and referrer headers
session = requests.Session()
session.headers.update({"User-Agent": user_agent, "Referer": referrer})
# Make a GET request to the website url and print the status code
response = session.get(website_url)
print(f"Visit {i+1}: Status code {response.status_code}")
# Wait for a random time between 1 and 5 seconds before the next visit
time.sleep(random.randint(1, 5))
# Load the website url in the driver and maximize the window
driver.get(website_url)
driver.maximize_window()
# Loop through the number of impressions per visit
for j in range(num_impressions):
# Scroll to a random position on the page using JavaScript
scroll_position = random.randint(0, driver.execute_script("return document.body.scrollHeight"))
driver.execute_script(f"window.scrollTo(0, {scroll_position})")
# Wait for a random time between 2 and 10 seconds before scrolling again
time.sleep(random.randint(2, 10))
# Print the current impression number and scroll position
print(f"Impression {j+1}: Scroll position {scroll_position}")
# Close the driver after finishing all impressions for this visit
driver.close()
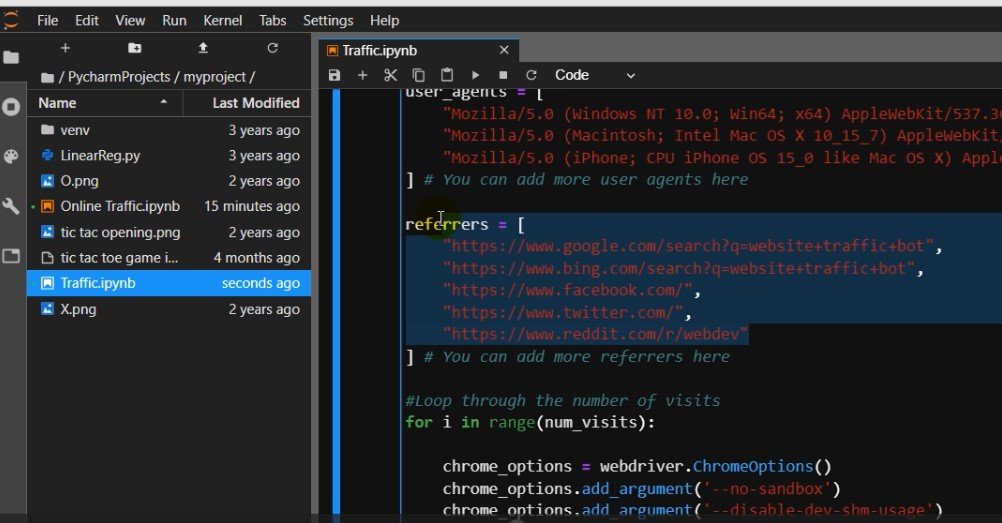
Here are some useful links related to the code and concepts used in the script:
- Python Requests Library Documentation: https://docs.python-requests.org/en/latest/
- Python Random Module Documentation: https://docs.python.org/3/library/random.html
- Python Time Module Documentation: https://docs.python.org/3/library/time.html
- Selenium Python Bindings Documentation: https://selenium-python.readthedocs.io/
- ChromeDriver Documentation: https://sites.google.com/a/chromium.org/chromedriver/home
- JavaScript scrollTo() Method Documentation: https://www.w3schools.com/jsref/met_win_scrollto.asp
These links can provide additional information on the libraries, modules, and methods used in the script, as well as related concepts such as web scraping, browser automation, and HTTP requests.