If you’re looking to create currency converter in Python that can convert currency in real-time you’re in the right place! Here we’ll show Real Time Currency Converter in Python that asks user for input and output currency types and then converts the currency in real-time.
Step by Step Video for Real Time Currency Converter in Python with Output
Step 1: Install Required Libraries
Before we start coding we need to install the forex-python
library. We can do this by running the following command in our Jupyter Notebook cell:
Python Program to Handle Missing Values in Data
Program Solve N-Queen Problem in Python
!pip install forex-python
This will install forex-python
library that we’ll be using to get the current exchange rate.
Step 2: Import Required Libraries
Once we have forex-python
library installed we can import it into our program by adding following line to our Jupyter Notebook cell:
from forex_python.converter import CurrencyRates
This line will import CurrencyRates
class from the forex_python.converter
module.
Step 3: Ask User for Input
Now that we have necessary libraries imported we can start building currency converter program. We’ll start by asking the user for the input currency type and output currency type.
We can do this by using the input()
function like this:
from_currency = input("Enter the input currency type: ")
to_currency = input("Enter the output currency type: ")
Step 4: Convert Currency
Now that we have input and output currency types we can use the CurrencyRates
class to get the current exchange rate and convert the currency.
We can do this by creating an instance of the CurrencyRates
class like this:
c = CurrencyRates()
Then we can use get_rate()
method of the CurrencyRates
class to get the exchange rate like this:
exchange_rate = c.get_rate(from_currency, to_currency)
Finally we can multiply input amount by the exchange rate to get the output amount like this:
input_amount = float(input("Enter the input amount: "))
output_amount = input_amount * exchange_rate
This will prompt user to enter input amount and then multiply it by the exchange rate to get output amount.
Step 5: Display Output
Now that we have output amount we can display it to the user by using the print()
function like this:
print(f"{input_amount} {from_currency} is equal to {output_amount} {to_currency}")
This will display input amount, input currency type, output amount, and output currency type to user.
Step 6: Complete Code
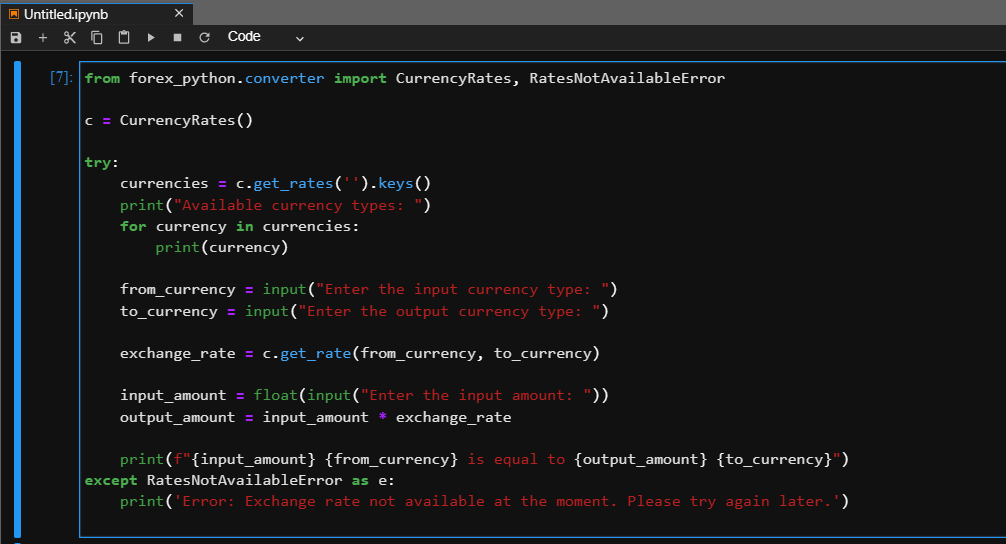
Here’s the complete code for our currency converter program:
pip install forex-python
from forex_python.converter import CurrencyRates, RatesNotAvailableError
c = CurrencyRates()
try:
currencies = c.get_rates('').keys()
print("Available currency types: ")
for currency in currencies:
print(currency)
from_currency = input("Enter the input currency type: ")
to_currency = input("Enter the output currency type: ")
exchange_rate = c.get_rate(from_currency, to_currency)
input_amount = float(input("Enter the input amount: "))
output_amount = input_amount * exchange_rate
print(f"{input_amount} {from_currency} is equal to {output_amount} {to_currency}")
except RatesNotAvailableError as e:
print('Error: Exchange rate not available at the moment. Please try again later.')
Here are some useful links related to the Python currency converter:
forex-python
library documentation: https://forex-python.readthedocs.io/en/latest/- Open Exchange Rates API documentation: https://docs.openexchangerates.org/
- Fixer.io API documentation: https://fixer.io/documentation
- Currency codes list: https://www.iso.org/iso-4217-currency-codes.html
These links provide more information about the forex-python
library, the data sources used for exchange rates, and the ISO codes for different currencies.