Step 1: Install Required Libraries
First we need to install gTTS library convert text to speech. You can install it using pip running following command:
!pip install gtts
Any Language Translator in Python
Write a Python Program to Detect Abusive Comment
Topic Identification System in Python
Step 2: Import Required Libraries
Next we need import gTTS library use gTTS class for text to-speech conversion. We also need to import IPython.display module play speech as audio file.
from gtts import gTTS
from IPython.display import Audio
Step 3: Convert Text to Speech
To Convert Text to Speech we need to create an instance of gTTS class and pass text as a string to its constructor. We can also specify language and speed of speech using optional parameters.
# Create a gTTS object and specify the text and language
tts = gTTS('Hello, World!', lang='en')
# Save the speech as an MP3 file
tts.save('hello.mp3')
# Play the speech as an audio file
Audio('hello.mp3', autoplay=True)
In this example we create a gTTS object and specify text as “Hello, World!” and the language as English. We then save the speech MP3 file and play it as audio file using Audio function.
Step 4: Using Text from a File
We can also convert text from file to speech using gTTS library. We read text from a file using Python’s built-in open function and pass it gTTS constructor.
# Read the text from a file
with open('sample.txt', 'r') as f:
text = f.read()
# Create a gTTS object and specify the text and language
tts = gTTS(text, lang='en')
# Save the speech as an MP3 file
tts.save('output.mp3')
# Play the speech as an audio file
Audio('output.mp3', autoplay=True)
In this example we read text from file named “sample.txt” using open function and store it in text variable. We then create gTTS object and pass the text variable to its constructor along with the language parameter. We save the speech as an MP3 file and play it as an audio file using the Audio function.
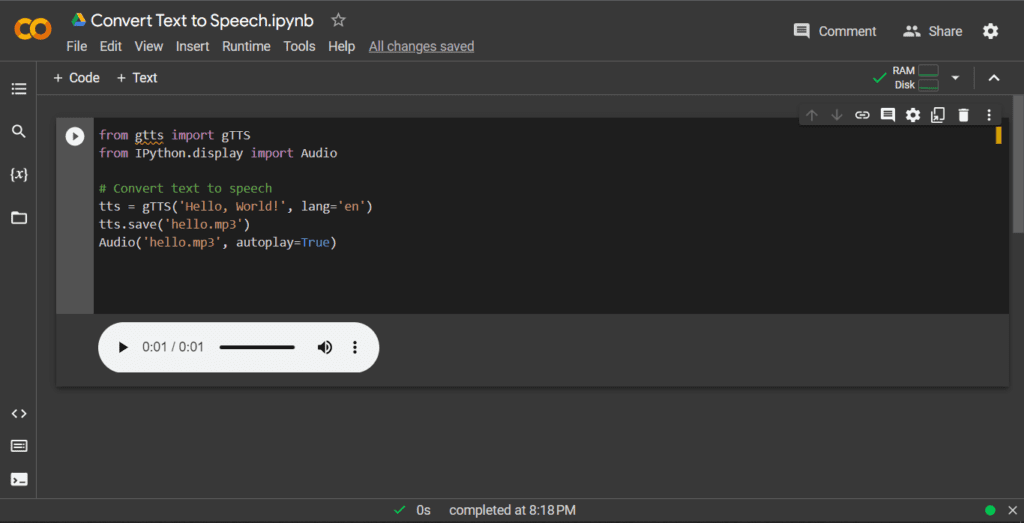
Complete Code
Here’s complete code that demonstrates how convert text to speech using Python and gTTS
library:
from gtts import gTTS
from IPython.display import Audio
# Convert text to speech
tts = gTTS('Hello, World!', lang='en')
tts.save('hello.mp3')
Audio('hello.mp3', autoplay=True)
In this code we first convert text “Hello, World!” to speech and play it as an audio file. Then convert text from file named “sample.txt” to speech and play it audio file. You can modify code to use your own text and files.
Here are some useful links related text-to-speech conversion using Python and gTTS
library:
- Official
gTTS
library documentation: https://gtts.readthedocs.io/en/latest/ gTTS
tutorial on RealPython: https://realpython.com/python-text-to-speech/- Tutorial on converting text to speech using Python: https://www.geeksforgeeks.org/convert-text-speech-python/
gTTS
GitHub repository: https://github.com/pndurette/gTTSgTTS
library on PyPI: https://pypi.org/project/gTTS/