Are you looking for Python Program to Convert any Video into MP3? Look no further than this Python program! With just a few lines of code you can quickly and easily convert any video file into an MP3 audio file.
The program uses popular MoviePy library to handle video processing allowing for seamless conversion from any format including MP4, AVI, MOV, WMV, FLV, and MKV. The program also includes a user-friendly GUI built with the Tkinter library, making it easy to navigate and select your video files.
To get started simply open program and select the video file you want to convert by clicking the “Browse” button. Once you’ve selected your file, click the “Convert to MP3” button and let the program do the rest. The program will extract the audio from the video file and save it as an MP3 file in the same directory as the original video.
Video steps of Python Program to Convert any Video into MP3
Also Learn:
URL Shortener App in Python with Output
Python Program to Download Youtube Videos
This Python program is a great tool for anyone looking to convert their video files into MP3s quickly and easily. Whether you need to convert a single file or an entire library this program has got you covered. Plus, with its lightweight and user-friendly design, it’s a breeze to use and won’t bog down your system.
If you have a video file that you’d like to convert to MP3 format you can use Python to do it easily. With a few lines of code, you can convert your video to an audio file without any hassle.
Step 1: Install Required Libraries
Before you can use the Python program you’ll need to install two libraries: tkinter and moviepy. You can install them using pip by running the following commands:
pip install tkinter
pip install moviepy
Step 2: Import Required Libraries
Once you’ve installed required libraries you can import them in your Python program using the following code:
from tkinter import *
from tkinter import filedialog
from tkinter import messagebox
from moviepy.editor import *
The tkinter library is used to create graphical user interface (GUI) for the program, while the moviepy library is used to work with video and audio files.
Step 3: Define Functions for File Selection and Conversion
To convert video to MP3 you’ll need to select video file and then convert it to an audio file. You can define two functions to do this: one for selecting the video file and another for converting it to MP3.
# Define a function to browse for a video file
def browse_file():
filename = filedialog.askopenfilename(initialdir="/", title="Select a File", filetypes=(("Video files", "*.mp4 *.avi *.mov *.wmv *.flv *.mkv"), ("all files", "*.*")))
if filename:
entry_filename.delete(0, END)
entry_filename.insert(0, filename)
# Define a function to convert the video to MP3
def convert_to_mp3():
# Get the filename from the entry widget
filename = entry_filename.get()
# Load the video file
video = VideoFileClip(filename)
# Extract the audio
audio = video.audio
# Define the output filename
output_filename = filename.split(".")[0] + ".mp3"
# Write the audio to the output file
audio.write_audiofile(output_filename)
# Close the video and audio files
video.close()
audio.close()
# Display a message box to show that the conversion is complete
messagebox.showinfo("Conversion Complete", f"The MP3 file '{output_filename}' has been created.")
The browse_file
function uses filedialog
module from tkinter to open a dialog box where you can select the video file. The function then inserts the filename into an entry widget in the GUI.
The convert_to_mp3
function does actual conversion. It loads the video file using the VideoFileClip
function from moviepy, extracts the audio using the audio
attribute and then writes the audio to an MP3 file using the write_audiofile
method. Finally function displays a message box to confirm that conversion is complete.
Step 4: Create the GUI
With the functions defined you can now create GUI for the program. You’ll need to create a window add widgets for selecting the video file and converting it to MP3 and define the layout of the widgets.
# Create the GUI
root = Tk()
root.title("Video to MP3 Converter")
root.geometry("400x200")
# Add a label and entry widget to enter the filename
label_filename = Label(root, text="Select a video file:")
entry_filename.pack(padx=10, pady=5)
button_browse = Button(root, text="Browse", command=browse_file)
button_browse.pack(pady=5)
We also add a button called “Browse” that when clicked opens a file dialog box that allows the user to select a video file.
button_browse = Button(root, text="Browse", command=browse_file)
button_browse.pack(pady=5)
The browse_file
function uses filedialog
module to create the dialog box. Once the user selects a file path is inserted into the entry widget.
def browse_file():
filename = filedialog.askopenfilename(initialdir="/", title="Select a File", filetypes=(("Video files", "*.mp4 *.avi *.mov *.wmv *.flv *.mkv"), ("all files", "*.*")))
if filename:
entry_filename.delete(0, END)
entry_filename.insert(0, filename)
The last element we add to GUI is a button called “Convert to MP3”. When this button is clicked, the convert_to_mp3
function is called.
button_convert = Button(root, text="Convert to MP3", command=convert_to_mp3)
button_convert.pack(pady=10)
The convert_to_mp3
function loads video file extracts the audio, defines the output filename, and writes the audio to a file. Finally a message box is displayed to inform the user that the conversion is complete.
def convert_to_mp3():
# Get the filename from the entry widget
filename = entry_filename.get()
# Load the video file
video = VideoFileClip(filename)
# Extract the audio
audio = video.audio
# Define the output filename
output_filename = filename.split(".")[0] + ".mp3"
# Write the audio to the output file
audio.write_audiofile(output_filename)
# Close the video and audio files
video.close()
audio.close()
# Display a message box to show that the conversion is complete
messagebox.showinfo("Conversion Complete", f"The MP3 file '{output_filename}' has been created.")
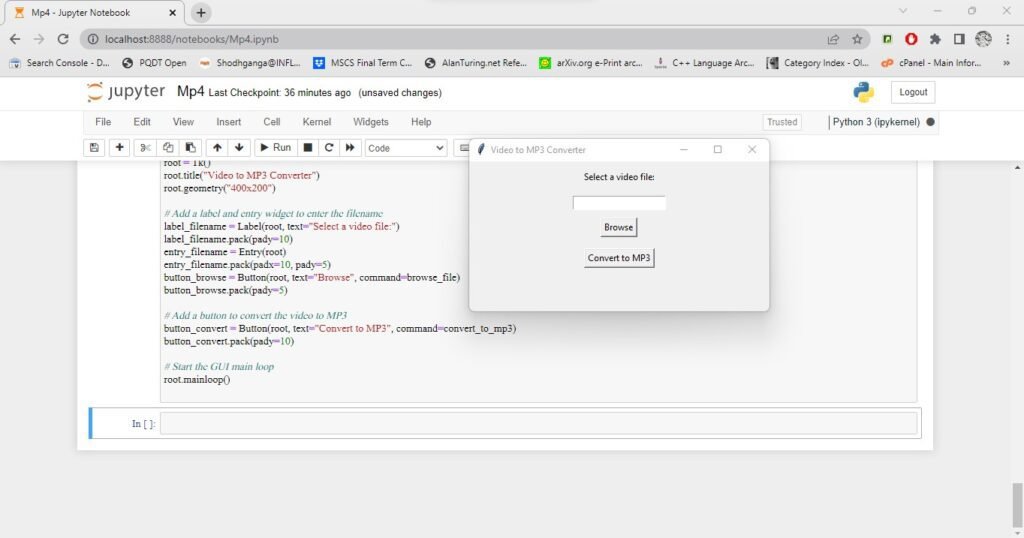
Complete Code
from tkinter import *
from tkinter import filedialog
from tkinter import messagebox
from moviepy.editor import *
# Define a function to browse for a video file
def browse_file():
filename = filedialog.askopenfilename(initialdir="/", title="Select a File", filetypes=(("Video files", "*.mp4 *.avi *.mov *.wmv *.flv *.mkv"), ("all files", "*.*")))
if filename:
entry_filename.delete(0, END)
entry_filename.insert(0, filename)
# Define a function to convert the video to MP3
def convert_to_mp3():
# Get the filename from the entry widget
filename = entry_filename.get()
# Load the video file
video = VideoFileClip(filename)
# Extract the audio
audio = video.audio
# Define the output filename
output_filename = filename.split(".")[0] + ".mp3"
# Write the audio to the output file
audio.write_audiofile(output_filename)
# Close the video and audio files
video.close()
audio.close()
# Display a message box to show that the conversion is complete
messagebox.showinfo("Conversion Complete", f"The MP3 file '{output_filename}' has been created.")
# Create the GUI
root = Tk()
root.title("Video to MP3 Converter")
root.geometry("400x200")
# Add a label and entry widget to enter the filename
label_filename = Label(root, text="Select a video file:")
label_filename.pack(pady=10)
entry_filename = Entry(root)
entry_filename.pack(padx=10, pady=5)
button_browse = Button(root, text="Browse", command=browse_file)
button_browse.pack(pady=5)
# Add a button to convert the video to MP3
button_convert = Button(root, text="Convert to MP3", command=convert_to_mp3)
button_convert.pack(pady=10)
# Start the GUI main loop
root.mainloop()
Here are some useful links related to video and audio processing in Python:
- MoviePy documentation: https://zulko.github.io/moviepy/
- PyDub documentation: https://github.com/jiaaro/pydub
- OpenCV documentation: https://opencv.org/
- FFmpeg documentation: https://ffmpeg.org/documentation.html
- Python Imaging Library (Pillow) documentation: https://pillow.readthedocs.io/en/stable/
Additionally, here are some related articles and tutorials that you may find helpful:
“Audio Processing in Python Part I: Sampling, Nyquist, and the Fast Fourier Transform” by Chris Cannam: https://www.researchgate.net/publication/236129729_Audio_Processing_in_Python_Part_I_Sampling_Nyquist_and_the_Fast_Fourier_Transform
“Extracting frames from video using OpenCV and Python” by Madhav Sainanee: https://towardsdatascience.com/extracting-frames-from-video-using-opencv-python-558d2ebe2b93
I hope these resources are helpful to you!