How do you reverse a list in Python? Explain with programming examples.
There are several ways to reverse a list in Python including
- Using [::-1] slicing syntax
- Using reversed() function
- Using list.reverse() method
- Using for loop to traverse the list in reverse order and building a new list
- Using sorted() function with the reverse argument set to True
- Using reduce() function from the functools module
- Using itertools.chain and reversed functions from the itertools module
Each of these methods has its own advantages and disadvantages so it is important to choose the one that best fit in particular situation and requirements.
Explore More Programming Challenges:
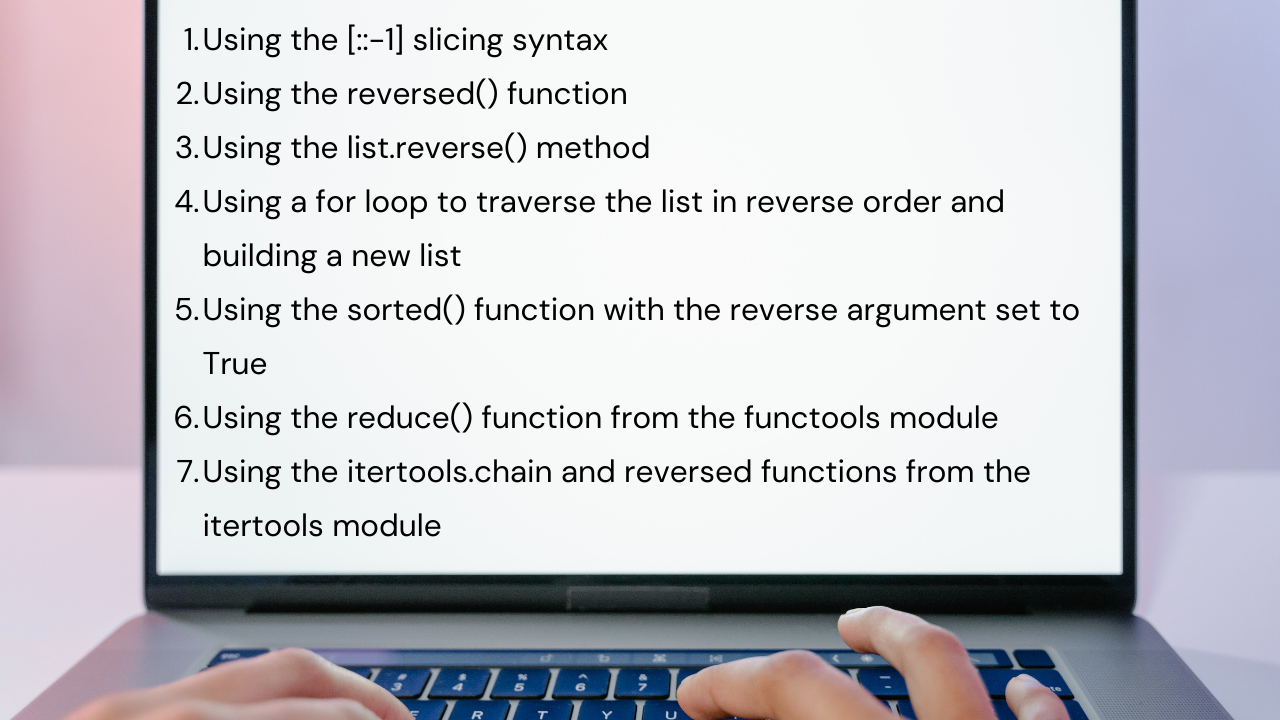
Reverse a List in Python Using the [::-1] slicing syntax:
# original list
list = [1, 2, 3, 4, 5]
# reversing the list using slicing
reversed_list = list[::-1]
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Reverse a List in Python Using the reversed() function:
# list
list = [1, 2, 3, 4, 5]
# reversed list using the reversed function
reversed_list = list(reversed(list))
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Reverse a List in Python Using the list.reverse() method:
# list
list = [1, 2, 3, 4, 5]
# reversing the list using the list.reverse method
list.reverse()
print(list) # Output: [5, 4, 3, 2, 1]
Reverse a List in Python Using a for loop:
# original list
list = [1, 2, 3, 4, 5]
# reversing the list using a for loop
reversed_list = []
for i in range(len(list)-1, -1, -1):
reversed_list.append(list[i])
print(reversed_list) # Output: [5, 4, 3, 2, 1]
Reverse a List in Python Using the sorted() function with the reverse argument set to True
# original list
list = [1, 2, 3, 4, 5]
# reversing the list using sorted() with reverse argument set to True
reversed_list = sorted(list, reverse=True)
print(reversed_list) # Output: [5, 4, 3, 2, 1]
The sorted() function takes the original list as argument and returns a new sorted list. By setting the reverse argument to True you can sort the list in reverse order. Note that this method creates new list so if you need to modify the original list in place you should use a different method such as the list.reverse() method or a for loop.
Reverse a List in Python Using the reduce() function from the functools module
from functools import reduce
lst = [1, 2, 3, 4, 5]
reversed_lst = reduce(lambda x, y: [y] + x, lst, [])
print(reversed_lst) # Output: [5, 4, 3, 2, 1]
Reverse a list in python Using the itertools.chain and reversed functions from the itertools module
import itertools
lst = [1, 2, 3, 4, 5]
reversed_lst = list(itertools.chain(reversed(lst)))
print(reversed_lst) # Output: [5, 4, 3, 2, 1]
In the above code the reversed()
function returns an iterator that generates the items of the input list in reverse order. The itertools.chain()
function chains the elements of this iterator to produce a single sequence of reversed elements. Finally the list()
function is used to convert the chained sequence into a list.